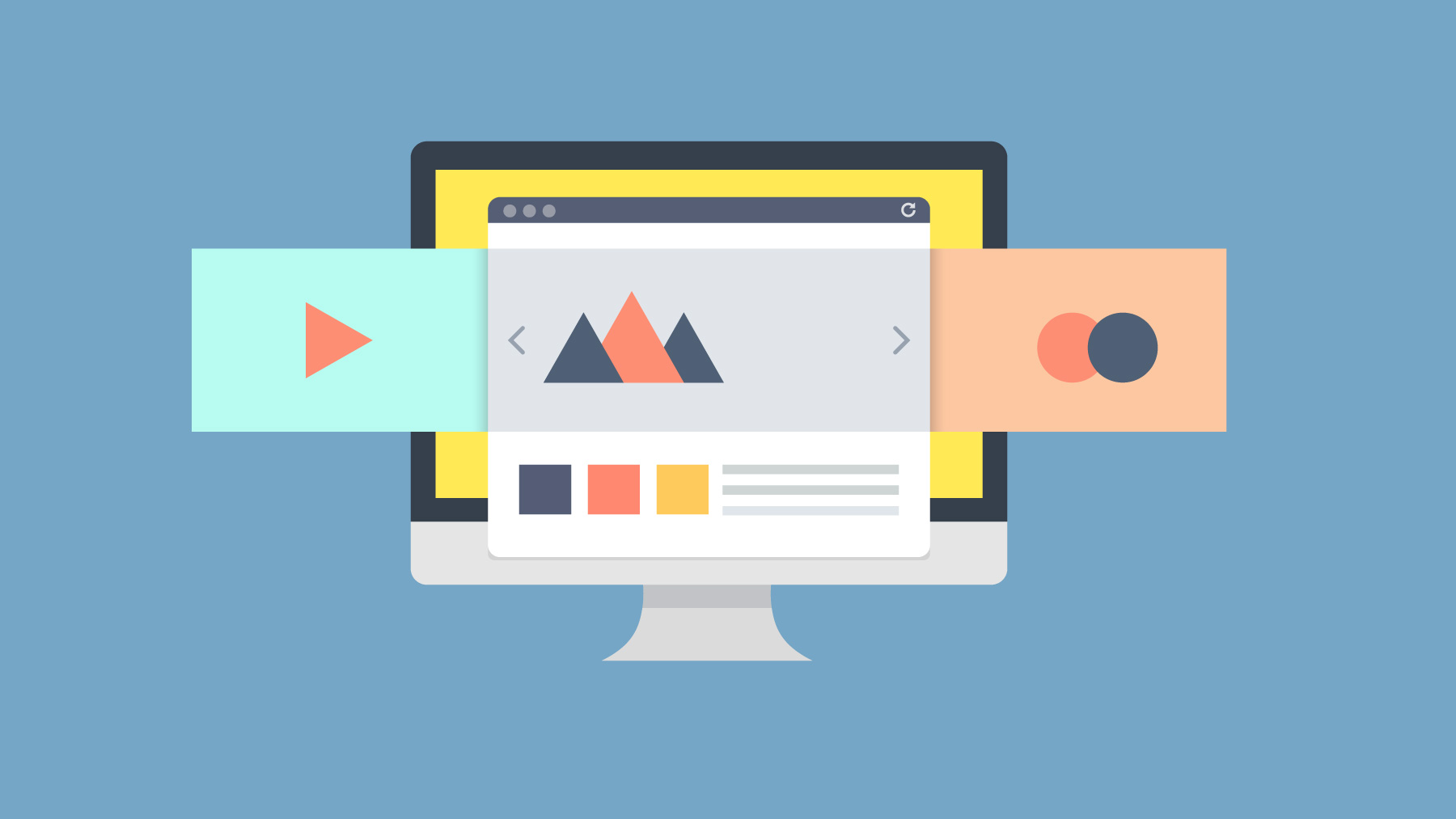
Updated to add ES6 compatibility and support for all modern browsers.
Carousels, sliders, slideshows — whatever you want to call them — they’re all over the web. Based on the premise of limited space with multiple messages to deliver, carousels are used to showcase services, help sell products, and capture the attention of new visitors.
In this article, we take a look at several approaches of JavaScript sliders and image carousels.
Tiniest JavaScript Slider
This quick tutorial will show you how to create a basic slider in less than 100 lines. The actual JavaScript is less than 30 lines 😊
<div class="slider">
<div class="slide" data-background="https://evermotion.org/files/model_images/3e0dbe0ce65face2b9d44ab7f013762d499867ed.jpg"></div>
<p class="caption caption-0">
The first slide.
<br><small>This is an awesome forest.</small>
</p>
<div class="slide" data-background="https://www.cbc.ca/natureofthings/content/images/episodes/talktalk_1920.jpg"></div>
<p class="caption caption-1">
The second slide, yay!
<br><small>This is a marvellous forest.</small>
</p>
<div class="slide" data-background="https://cdn1.epicgames.com/ue/product/Screenshot/MAWIRedwoodForestPackV2Screenshot0001-1920x1080-e62973805ee13fbc3bca02a2ade9e9c4.jpg"></div>
<p class="caption caption-2">
Oh look, a third slide!
<br><small>This is a bloody brilliant forest. It's CGI.</small>
</p>
</div>
JavaScript
const slideArray = [];
for (let i = 0; i < document.querySelectorAll('.slider div').length; i++) {
slideArray.push(document.querySelectorAll('.slider div')[i].dataset.background);
}
let currentSlideIndex = -1;
function advanceSliderItem() {
currentSlideIndex++;
if (currentSlideIndex >= slideArray.length) {
currentSlideIndex = 0;
}
document.querySelector('.slider').style.cssText = 'background: url("' + slideArray[currentSlideIndex] + '") no-repeat center center; background-size: cover;';
const elems = document.getElementsByClassName('caption');
for (let i = 0; i < elems.length; i++) {
elems[i].style.cssText = 'opacity: 0;';
}
const currentCaption = document.querySelector('.caption-' + (currentSlideIndex));
currentCaption.style.cssText = 'opacity: 1;';
}
let intervalID = setInterval(advanceSliderItem, 3000);
CSS
.slider {
position: relative;
overflow: hidden;
width: 100%;
height: 300px;
margin: 48px auto;
background-size: cover;
border-radius: 3px;
box-shadow: 0 0 16px rgba(0, 0, 0, 0.1);
transition: background-image 0.8s ease-in-out;
}
.slider .slide {
display: none;
}
.caption {
position: absolute;
right: 0;
top: 0;
bottom: 0;
opacity: 0;
display: inline-block;
padding: 48px;
background: rgba(0, 0, 0, 0.75);
color: white;
margin: 0;
font-size: 24px;
font-weight: 700;
transition: all 0.8s ease-in-out;
}
.caption small {
font-size: 16px;
font-weight: 400;
}
See a demo here.
More Approachable Vanilla JS Sliders
The past weeks had me hunting for the fastest JavaScript sliders out there for a secret project, and I have documented my findings and my experiments below.
Tiny Slider 2 – Vanilla JavaScript Slider (with swipe support)
Tiny Slider 2 is a great slider replacement for any jQuery-powered slider out there. Small, fast and feature-packed. Tiny Slider is inspired by Owl Carousel.
Supernova Slider – Vanilla JavaScript Swipe Slider (with swipe support)
A small slider for fast pages, with no bells and whistles, but with mobile support.
Supernova Slider – Vanilla JavaScript Slider (no swipe support)
A small slider for fast pages on modern browsers, with no bells and whistles, Flexbox CSS and full ES6 compatibility.
Supernova Slider – Sticks & Stones (no swipe support)
The smallest Vanilla JavaScript (ES6) slider, suitable for landing pages, with no bells and whistles.