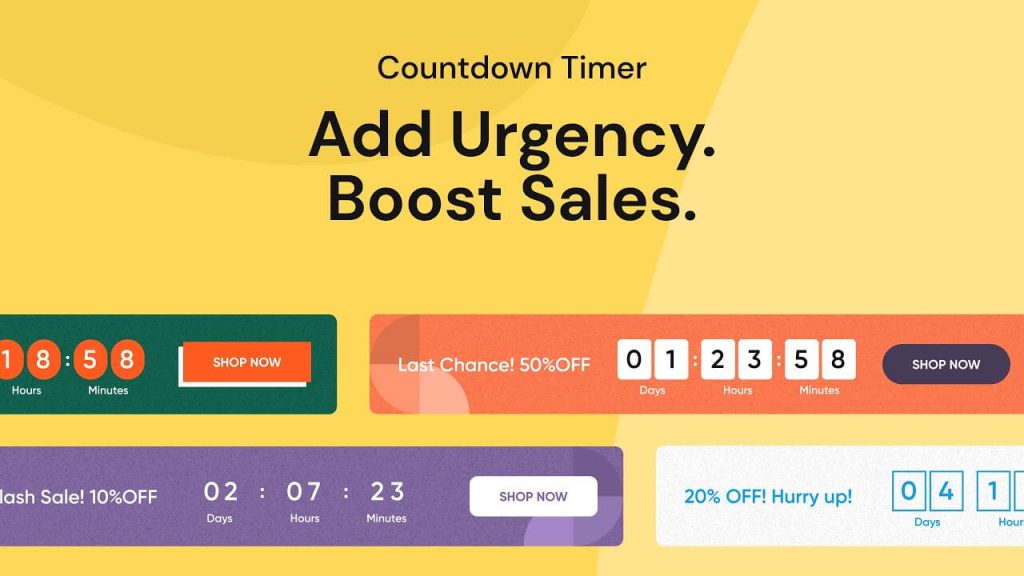
You can (probably) see this top bar right now, with a nice 30% off sale for my WordPress Lighthouse plugin.
And this is how I built it, without using any eCommerce plugin. The only thing is that you need to calculate the number of days until your sales end and manually add it to the code. The advantage of this code is that it’s extremely fast and all the code can be inlined.

Let’s start with the HTML structure:
<div class="banner-promo">
<div class="wrapper--1100">
<div class="banner-promo__coupon"><a href="https://getbutterfly.com/wordpress-plugins/lighthouse/">30% Off</a></div>
<div class="banner-promo__text">Hurry up, <a href="https://getbutterfly.com/wordpress-plugins/lighthouse/">the offer is only valid</a> until <span class="has-style-bold">December 15, 2021</span>!</div>
<ul id="countdown">
<li><span id="day" class="numbers">00</span><p class="name">days</p></li>
<li><span id="hour" class="numbers">00</span><p class="name">hours</p></li>
<li><span id="minute" class="numbers">00</span><p class="name">minutes</p></li>
<li><span id="second" class="numbers">00</span><p class="name">seconds</p></li>
</ul>
</div>
</div>
The bar is split into 3 parts, the coupon value (or discount), the text and the actual countdown.
Next, we need to style this bar. If you want it to look like mine, here’s the code:
.banner-promo {
background-color: #ffd147;
padding: 1rem 0;
width: 100%;
}
.banner-promo .wrapper--1100 {
display: flex;
flex-direction: row;
align-items: center;
justify-content: center;
}
.wrapper--1100 {
max-width: 1100px;
margin-right: auto;
margin-left: auto;
}
.banner-promo__coupon {
display: inline-block;
font-weight: 500;
font-size: 1.375rem;
color: #fff;
text-transform: uppercase;
padding: 0.5rem 1.1875rem;
background: #172153;
border-radius: 48px;
}
.banner-promo__coupon a {
color: #ffffff;
}
.banner-promo__text {
display: inline-block;
margin: 0 2.5rem;
font-size: 1.125rem;
color: #0c0f1f;
}
.has-style-bold {
font-weight: 700;
}
ul#countdown {
display: flex;
list-style-type: none;
margin: 0;
padding: 0;
}
ul#countdown li {
display: flex;
flex-direction: column;
margin-right: 4px;
width: 72px;
height: 64px;
padding: 0.625rem 0.875rem;
background-color: #fff;
border-radius: 8px;
justify-content: center;
align-items: center;
}
ul#countdown .numbers {
display: block;
font-size: 24px;
line-height: 1;
}
ul#countdown .name {
font-size: 10px;
margin: 0;
}
That’s it for structure and styling. We need to make the countdown work now:
document.addEventListener('DOMContentLoaded', () => {
if (document.getElementById('day')) {
const deadline = new Date(Date.now() + 20 * 24 * 3600 * 1000); // 20 days from now
let x = setInterval(() => {
let now = Date.now(),
t = deadline - now,
days = Math.floor(t / (1000 * 60 * 60 * 24)),
hours = Math.floor((t % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60)),
minutes = Math.floor((t % (1000 * 60 * 60)) / (1000 * 60)),
seconds = Math.floor((t % (1000 * 60)) / 1000);
document.getElementById('day').innerHTML = days ;
document.getElementById('hour').innerHTML = hours;
document.getElementById('minute').innerHTML = minutes;
document.getElementById('second').innerHTML = seconds;
if (t < 0) {
clearInterval(x);
document.getElementById('day').innerHTML = '0';
document.getElementById('hour').innerHTML = '0';
document.getElementById('minute').innerHTML = '0' ;
document.getElementById('second').innerHTML = '0';
}
}, 1000);
}
});
There’s a small caveat here, only the days get updated, but this was enough for my specific case study.
That’s all there is to it!
In my example, I have also added a condition to only show the countdown if between specific dates. Something like below (again, note that I didn’t need minutes or seconds):
<?php
function dateIsInBetween(\DateTime $from, \DateTime $to, \DateTime $subject) {
return $subject->getTimestamp() > $from->getTimestamp() && $subject->getTimestamp() < $to->getTimestamp() ? true : false;
}
$today = new \DateTime('now');
$dateFrom = new \DateTime('2021-11-24');
$dateTo = new \DateTime('2022-01-15');
if (dateIsInBetween($dateFrom, $dateTo, $today)) {
// Display the countdown
}
This should be easy enough to get you started and build a nice countdown bar.