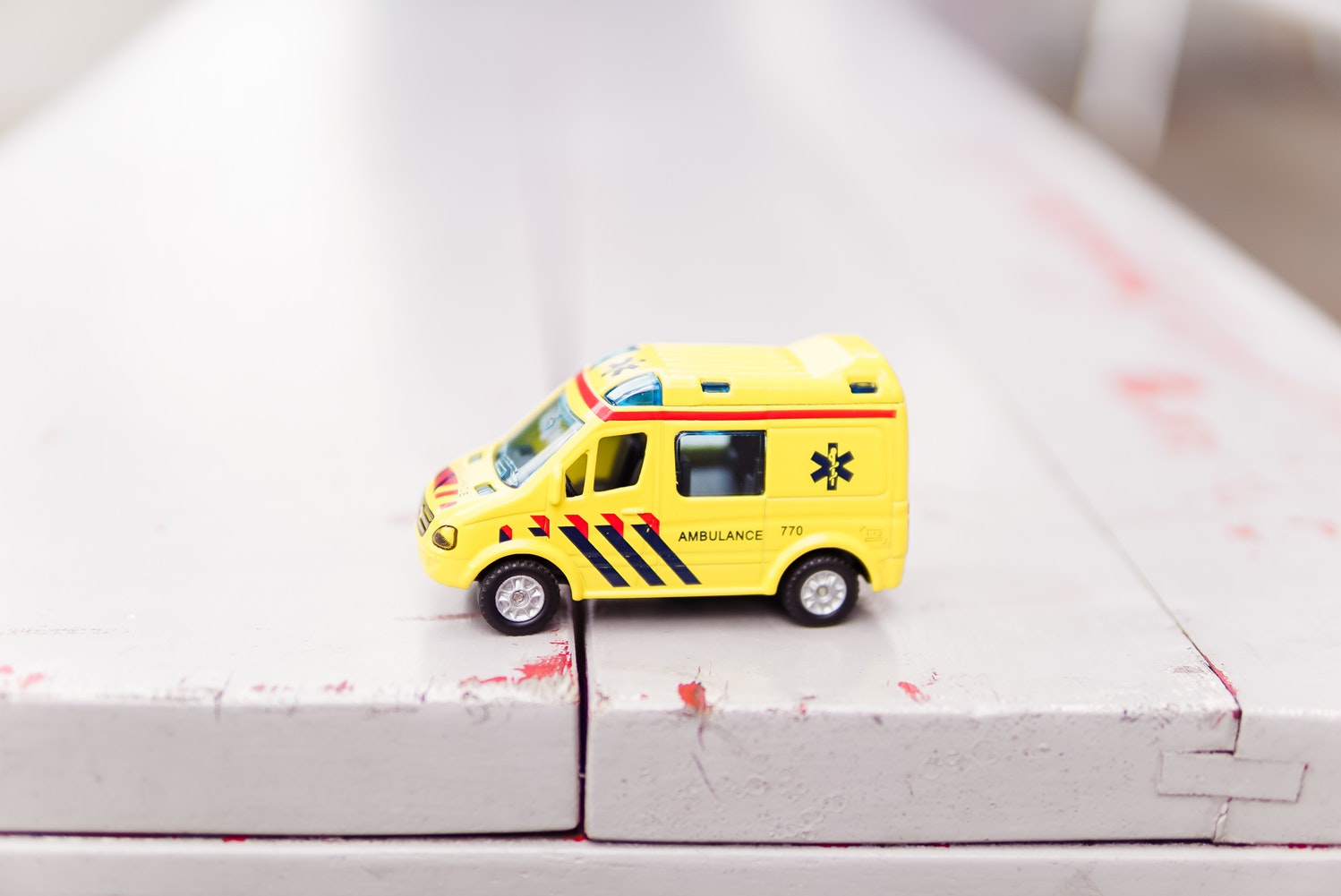
Internal, client-oriented plugins are mostly used by maintenance agencies. They supply a plugin to their clients, allowing them to submit assistance requests straight from their WordPress dashboard. The email can then be plugged into a CRM or into a support system. You will also want to get the client’s technical details.
How do you code such a plugin, though? Well, keep reading and you’ll see how easy it is.
The plugin structure is as follows:
wp-assistance-request
┝ includes/
┝ functions.php
┝ settings.php
┝ wp-assistance-request.php
First of all, in your main plugin file – wp-assistance-request.php
– add the code below:
/**
* Include plugin settings
*/
include 'includes/functions.php';
include 'includes/settings.php';
/**
* Create plugin options menu
*/
function wpd_plugin_menu() {
add_menu_page(__('WPDublin', 'wpdublin'), __('WPDublin', 'wpdublin'), 'manage_options', 'wpd_settings', 'wpd_settings', 'dashicons-admin-tools', 4);
}
add_action('admin_menu', 'wpd_plugin_menu');
Your functions.php
file will look like this:
function wpd_get_site_details() {
global $wpdb, $wp_version;
// Assign details
$wpd_current_theme = wp_get_theme();
$wpd_has_gzip = 0;
if ($_SERVER['HTTP_ACCEPT_ENCODING'] == 'gzip' || function_exists('ob_gzhandler') || ini_get('zlib.output_compression')) {
$wpd_has_gzip = 1;
}
$wpd_has_https = 'HTTPS=off';
if (isset($_SERVER['HTTPS']) && $_SERVER['HTTPS'] == 'on') {
$wpd_has_https = 'HTTPS=on';
} else if (!empty($_SERVER['HTTP_X_FORWARDED_PROTO']) && $_SERVER['HTTP_X_FORWARDED_PROTO'] == 'https' || !empty($_SERVER['HTTP_X_FORWARDED_SSL']) && $_SERVER['HTTP_X_FORWARDED_SSL'] == 'on') {
$wpd_has_https = 'HTTPS=on;SSL=on';
}
$wpd_db_size = 0;
$results = $wpdb->get_results("SHOW TABLE STATUS FROM " . DB_NAME, ARRAY_A);
foreach ($results as $row) {
$usedspace = $row['Data_length'] + $row['Index_length'];
$usedspace = $usedspace / 1024;
$usedspace = round($usedspace, 2);
$wpd_db_size += $usedspace;
}
$wpd_cpu = '';
if (function_exists('sys_getloadavg')) {
$load = sys_getloadavg();
$wpd_cpu = 'Current CPU load: ' . implode(', ', $load);
}
$wpd_wp_version = $wp_version; // WordPress version
$wpd_wp_theme = $wpd_current_theme->get('Name') . ' <code>' . $wpd_current_theme->get('Version') . '</code> (<code>' . get_template_directory_uri() . '</code>)'; // Current theme
$wpd_wp_memory = WP_MEMORY_LIMIT; // Memory limit
$wpd_wp_db_size = $wpd_db_size; // Database size
$wpd_server_version = $_SERVER['SERVER_SOFTWARE']; // Server software
$wpd_server_protocol = wp_get_server_protocol(); // Server protocol
$wpd_server_ssl = $wpd_has_https; // Server certificate
$wpd_server_gzip = $wpd_has_gzip; // GZip
$wpd_server_cpu = $wpd_cpu; // CPU load
$wpd_php_version = PHP_VERSION; // PHP version
$wpd_mysql_version = $wpdb->db_version(); // MySQL version
$wpd_active_plugins_array = get_option('active_plugins');
$wpd_active_plugins = '<ul>';
foreach($wpd_active_plugins_array as $key => $value) {
$string = explode('/', $value);
$wpd_active_plugins .= '<li>' . $string[0] . '</li>';
}
$wpd_active_plugins .= '</ul>';
$specificationsTable = '<h3>Site Specifications</h3>
<ul>
<li>WordPress Address (URL): ' . get_option('siteurl') . '</li>
<li>Site Address (URL): ' . home_url() . '</li>
<li>WordPress version: <code>' . $wpd_wp_version . '</code></li>
<li>Current theme: ' . $wpd_wp_theme . '</li>
<li>WordPress memory limit: <code>' . $wpd_wp_memory . '</code></li>
<li>WordPress database size: <code>' . $wpd_wp_db_size . '</code></li>
<li>Server software: <code>' . $wpd_server_version . '</code></li>
<li>Server PHP version: <code>' . $wpd_php_version . '</code></li>
<li>Server MySQL version: <code>' . $wpd_mysql_version . '</code></li>
<li>Server protocol: <code>' . $wpd_server_protocol . '</code></li>
<li>Server certificate: <code>' . $wpd_server_ssl . '</code></li>
<li>Server GZip support: <code>' . $wpd_has_gzip . '</code></li>
<li>Server CPU: <code>' . $wpd_server_cpu . '</code></li>
<li>Active plugins: <code>' . $wpd_active_plugins . '</li>
</ul>';
/**
* What else you might need to get
*
* List of registered post types
* List of all plugins
* List of all themes
* Administrator email (subject to privacy/GDPR)
* HTTP/1 / HTTP/2 support
* OPcache support
* Is debug mode active
*/
return $specificationsTable;
}
function wpd_remote_addr() {
if (isset($_SERVER['HTTP_CF_CONNECTING_IP'])) {
$_SERVER['REMOTE_ADDR'] = $_SERVER['HTTP_CF_CONNECTING_IP'];
}
if (array_key_exists('HTTP_X_FORWARDED_FOR', $_SERVER)) {
$ip = ip2long($_SERVER['REMOTE_ADDR']);
}
return $_SERVER['REMOTE_ADDR'];
}
Your settings.php
file will look like this:
if (!defined('ABSPATH')) exit; // Exit if accessed directly
function wpd_settings() { ?>
<div class="wrap">
<h2><?php _e('WordPress Dublin', 'wpdublin'); ?></h2>
<?php $tab = isset($_GET['tab']) ? $_GET['tab'] : 'assistance'; ?>
<h2 class="nav-tab-wrapper">
<a href="<?php echo admin_url('admin.php?page=wpd_settings&tab=assistance'); ?>" class="nav-tab <?php echo $tab == 'assistance' ? 'nav-tab-active' : ''; ?>"><?php _e('Request Assistance', 'wpdublin'); ?></a>
</h2>
<?php if ((string) $tab === 'assistance') {
if (isset($_POST['info_update']) && current_user_can('manage_options')) {
$wpd_email_request_subject = sanitize_text_field($_POST['wpd_email_request_subject']);
$wpd_email_request_body = wp_kses_post($_POST['wpd_email_request_body']);
$headers = array('Content-Type: text/html; charset=UTF-8');
$wpd_body = '<h3>' . $wpd_email_request_subject . '</h3>' . $wpd_email_request_body;
$wpd_body .= wpd_get_site_details();
wp_mail('support@example.com', 'WPDublin Internal Support Request', $wpd_body, $headers);
echo '<div class="updated notice is-dismissible">
<p>' . __('Email request sent!', 'wpdublin') . '</p>
<p>' . __('We will investigate the issue and we will contact you if we need more details. Thank you for your patience.', 'wpdublin') . '</p>
</div>';
}
$settings = array('media_buttons' => true);
?>
<form method="post" action="">
<h3><?php _e('New Assistance Request', 'wpdublin'); ?></h3>
<p><span class="dashicons dashicons-editor-help"></span> <?php _e('What can we help you with today?', 'wpdublin'); ?></p>
<p>
<label for="wpd-email-request-subject"><?php _e('Subject', 'wpdublin'); ?></label><br>
<input type="text" name="wpd_email_request_subject" id="wpd-email-request-subject" placeholder="<?php _e('I need help with...', 'wpdublin'); ?>" class="regular-text">
<br><small><?php _e('Keep the request subject short and concise.', 'wpdublin'); ?></small>
</p>
<p>
<label for="wpd-email-request-priority">Priority</label><br>
<select name="wpd_email_request_priority" id="wpd-email-request-priority" class="regular-text">
<option value="0">Low</option>
<option value="1">Minor</option>
<option value="2">Normal</option>
<option value="3">Major</option>
<option value="4">Critical</option>
</select>
<br><small>Use it responsibly.</small>
</p>
<p>
<label for="wpd-email-request-body">Description/Request</label><br>
<?php wp_editor('', 'wpd_email_request_body', $settings); ?>
</p>
<p><input type="submit" name="info_update" class="button button-primary" value="<?php _e('Send Request', 'wpdublin'); ?>"></p>
</form>
<?php } ?>
</div>
<?php
}
That’s it, you now have a functional assistance request plugin for WordPress.