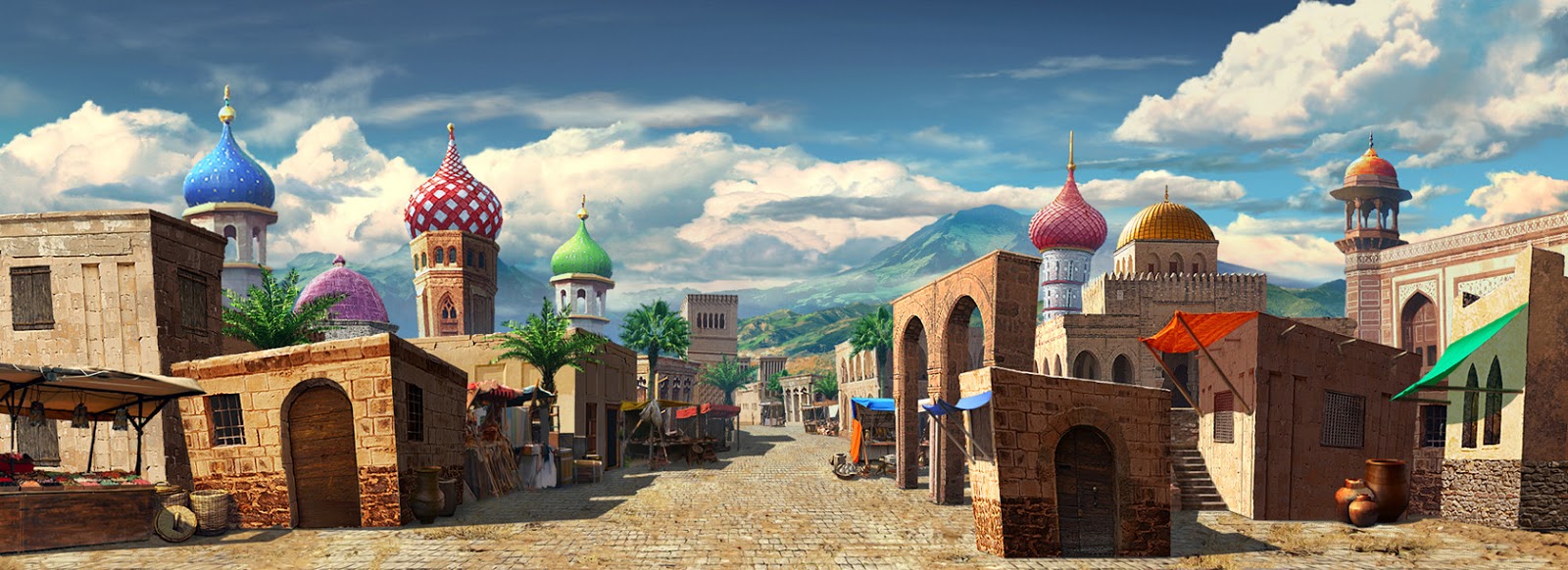
I’ve recently taken over a WordPress website, having a combination of bbPress and BuddyPress. Not my cup of tea, although I’ve used them both, with bbPress quite extensively years ago. I’ve since developed my own forum engine, an updated fork of the now defunct Mingle Forum, with support for Flex CSS, PHP 7.1+ and ES6. No Internet Explorer and no looking back.
For this project though, I had to move/migrate forums, topics, and replies to my Mingle Forum.
Without further ado, here’s the code I used:
/**
* Migration routine for bbPress to Mingle Forum
*/
$args = [
'post_type' => 'forum',
'posts_per_page' => -1,
'post_parent' => 0,
'order' => 'ASC'
];
$the_query = new WP_Query($args);
if ($the_query->have_posts()) {
while ($the_query->have_posts()) {
global $wpdb;
$the_query->the_post();
$fid = $the_query->post->ID;
// Step 1
echo '<p><code>Creating forum groups (categories)</code></p>';
$wpdb->query($wpdb->prepare('INSERT INTO wp_forum_groups (id, name) VALUES (%d, %s)', $fid, get_the_title($fid)));
$topic_arguments = [
'post_type' => 'forum',
'posts_per_page' => -1,
'post_parent' => $fid,
'order' => 'ASC'
];
$topic_query = new WP_Query($topic_arguments);
if ($topic_query->have_posts()) {
while($topic_query->have_posts()) {
$topic_query->the_post();
$fid = $topic_query->post->ID;
// Step 2
echo '<p><code>Populating forum groups (categories)</code></p>';
$wpdb->query($wpdb->prepare('INSERT INTO wp_forum_forums (id, name, parent_id) VALUES (%d, %s, %d)', $fid, get_the_title($fid), $topic_query->post->post_parent));
}
}
}
}
$topic_arguments = [
'post_type' => 'topic',
'posts_per_page' => -1,
'order' => 'ASC'
];
$topic_query = new WP_Query($topic_arguments);
if ($topic_query->have_posts()) {
while ($topic_query->have_posts()) {
global $wpdb;
$topic_query->the_post();
$fid = $topic_query->post->ID;
$author = $topic_query->post->post_author;
// Step 3
echo '<p><code>Populating forum threads (topics)</code></p>';
$wpdb->query($wpdb->prepare('INSERT INTO wp_forum_threads (id, parent_id, subject, date, starter, last_post) VALUES (%d, %d, %s, "' . $topic_query->post->post_date . '", ' . $author . ', "' . $topic_query->post->post_modified . '")', $fid, $topic_query->post->post_parent, get_the_title($fid)));
echo '<p><code>Populating forum threads (starters)</code></p>';
$wpdb->query($wpdb->prepare('INSERT INTO wp_forum_posts (id, text, parent_id, date, author_id, subject) VALUES (%d, %s, ' . $topic_query->post->post_parent . ', "' . $topic_query->post->post_date . '", ' . $author . ', %s)', $fid, $topic_query->post->post_content, get_the_title($fid)));
}
}
$topic_arguments = [
'post_type' => 'reply',
'posts_per_page' => -1,
'order' => 'ASC'
];
$topic_query = new WP_Query($topic_arguments);
if ($topic_query->have_posts()) {
while ($topic_query->have_posts()) {
global $wpdb;
$topic_query->the_post();
$fid = $topic_query->post->ID;
$author = $topic_query->post->post_author;
// Step 4
echo '<p><code>Populating forum posts (replies)</code></p>';
$wpdb->query($wpdb->prepare('INSERT INTO wp_forum_posts (id, text, parent_id, date, author_id, subject) VALUES (%d, %s, ' . $topic_query->post->post_parent . ', "' . $topic_query->post->post_date . '", ' . $author . ', %s)', $fid, $topic_query->post->post_content, get_the_title($fid)));
}
}
$threads = $wpdb->get_results("SELECT * FROM wp_forum_threads", ARRAY_A);
foreach ($threads as $thread_key => $thread_row) {
$id = $thread_row['id'];
$parent_id = $thread_row['parent_id'];
$subject = $thread_row['subject'];
$posts = $wpdb->get_results("SELECT * FROM wp_forum_posts WHERE parent_id = $id", ARRAY_A);
foreach ($posts as $post_key => $post_row) {
$wpdb->query("UPDATE wp_forum_posts SET parent_id = $id WHERE subject = '$subject'");
}
}
Notes
This migration process has been performed on bbPress 2 (latest version as of August 2019) and Mingle Forum (latest version — an updated version is coming soon).
Backup your database, or better yet, clone your site and perform the migration there. I broke my local clone six times.
Image credit: Shen Fei