This is the second Canvas visualization I’m experimenting with. The first one was a Julia fractal animation.
This article is part of the JavaScript Canvas series where I post experiments, JavaScript generative art, visualizations, Canvas animations, code snippets and how-to’s.
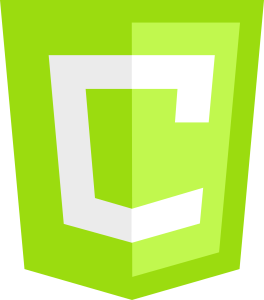
Here are some simple waves generated using a sine function and Math.PI
. Again, I won’t go into maths details or break down the code.
Here’s the JavaScript code:
document.addEventListener('DOMContentLoaded', () => {
let canvas = document.getElementById('canvas'),
ctx = canvas.getContext('2d'),
width = 500,
height = 500;
canvas.width = width;
canvas.height = height;
ctx.fillRect(0,0,canvas.width,canvas.height);
ctx.strokeStyle = '#badc58';
ctx.lineWidth = 2;
let waves = [];
for (let w =18; w > 10; w-=0.5) {
waves.push({amp : (w * 0.05), freq : 0, move : 0});
}
function render() {
ctx.fillRect(0, 0, canvas.width, canvas.height);
for(w = 0; w < waves.length; w++) {
let i = w + 16,
wave = waves[w];
ctx.beginPath();
ctx.moveTo(width, Math.sin(wave.freq * Math.PI / i));
for (let x = width; x >=0; x--) {
let y = Math.sin(wave.freq * Math.PI / i / 2);
wave.freq += wave.amp;
ctx.lineTo(x, (w * 32) + y * 15);
}
wave.move += (i * 0.1);
wave.freq = wave.move;
ctx.stroke();
}
requestAnimationFrame(render);
}
render();
});
Give it a whirl!