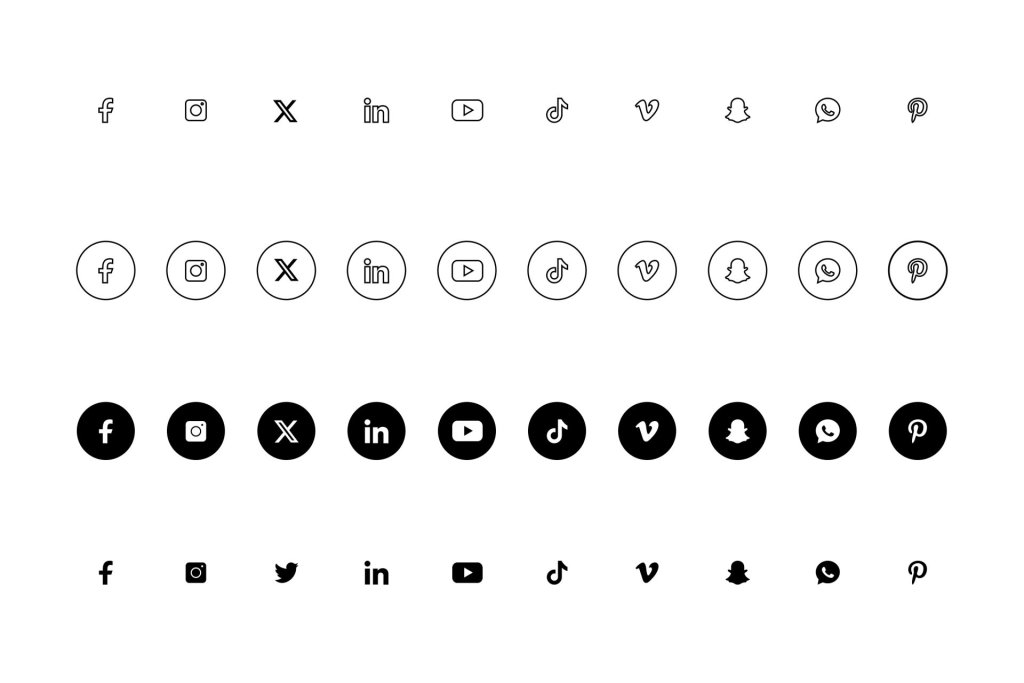
Social media links are such a common design element, and it’s now one less thing to think about when building a new website. No more icon libraries or grainy PNGs of social media logos.
One downside of the social links block is that there are no aria-
labels, and various SEO tools complain about this oversight. To accomplish this, we’re going to take advantage of the render_block_*
filter available in WordPress. By using the block name to build the filter, we’ll end up with something like this: add_filter( 'render_block_core/social-link', ... )
which allows us to filter the $block_content
– the actual HTML markup of the social link block as the page is rendering it.
Note that we’re filtering the core/social-link
block, not the core/social-links
block – that extra s
at the end is for the parent block that holds all the links in the row. We want to modify the inner block that contains the single link itself.
The general function will look something like this:
function filter_social_icons_block( $block_content, $block ) {
// Do stuff.
return $block_content;
}
add_filter( 'render_block_core/social-link', 'filter_social_icons_block', 10, 2 );
The filter also provides us with a second argument: $block
, the full block array, including the attribute values. This will be important because which social network service the block is rendering will be saved as an attribute value in their $block['attrs']['service']
. We check the service, looking for the social network we want to filter, and then swap out the URL.
To swap the actual URL, we’ll use the WP_HTML_Tag_Processor
to find the link and set the href
attribute:
if ( 'x' === $block['attrs']['service'] ) {
$new_content = new WP_HTML_Tag_Processor( $block_content );
if ( $new_content->next_tag( 'a' ) ) {
$new_content->set_attribute( 'aria-label', 'X (Twitter)' );
}
$block_content = $new_content->get_updated_html();
}
And that’s basically it! Let’s put it all together:
<?php
/**
* Filter the social icons block.
*
* @param string $block_content The block content.
* @param array $block The block.
*
* @return string
*/
function supernova_filter_social_icons_block( $block_content, $block ) {
if ( 'twitter' === $block['attrs']['service'] ) {
$new_content = new WP_HTML_Tag_Processor( $block_content );
if ( $new_content->next_tag( 'a' ) ) {
$new_content->set_attribute( 'aria-label', 'Twitter' );
}
$block_content = $new_content->get_updated_html();
}
if ( 'x' === $block['attrs']['service'] ) {
$new_content = new WP_HTML_Tag_Processor( $block_content );
if ( $new_content->next_tag( 'a' ) ) {
$new_content->set_attribute( 'aria-label', 'X' );
}
$block_content = $new_content->get_updated_html();
}
if ( 'facebook' === $block['attrs']['service'] ) {
$new_content = new WP_HTML_Tag_Processor( $block_content );
if ( $new_content->next_tag( 'a' ) ) {
$new_content->set_attribute( 'aria-label', 'Facebook' );
}
$block_content = $new_content->get_updated_html();
}
if ( 'instagram' === $block['attrs']['service'] ) {
$new_content = new WP_HTML_Tag_Processor( $block_content );
if ( $new_content->next_tag( 'a' ) ) {
$new_content->set_attribute( 'aria-label', 'Instagram' );
}
$block_content = $new_content->get_updated_html();
}
if ( 'linkedin' === $block['attrs']['service'] ) {
$new_content = new WP_HTML_Tag_Processor( $block_content );
if ( $new_content->next_tag( 'a' ) ) {
$new_content->set_attribute( 'aria-label', 'LinkedIn' );
}
$block_content = $new_content->get_updated_html();
}
return $block_content;
}
add_filter( 'render_block_core/social-link', 'supernova_filter_social_icons_block', 10, 2 );
The options here are pretty open. You could use this method to add a CSS class or force links to open in a new tab. You could show different social link URLs in different situations, for example swapping in an author’s personal X (Twitter) link on a single blog post template. Just remember that the filter only runs on the front of your website, not in the block editor, so anything you do with it will not show up inside the editor, only on the final page render.