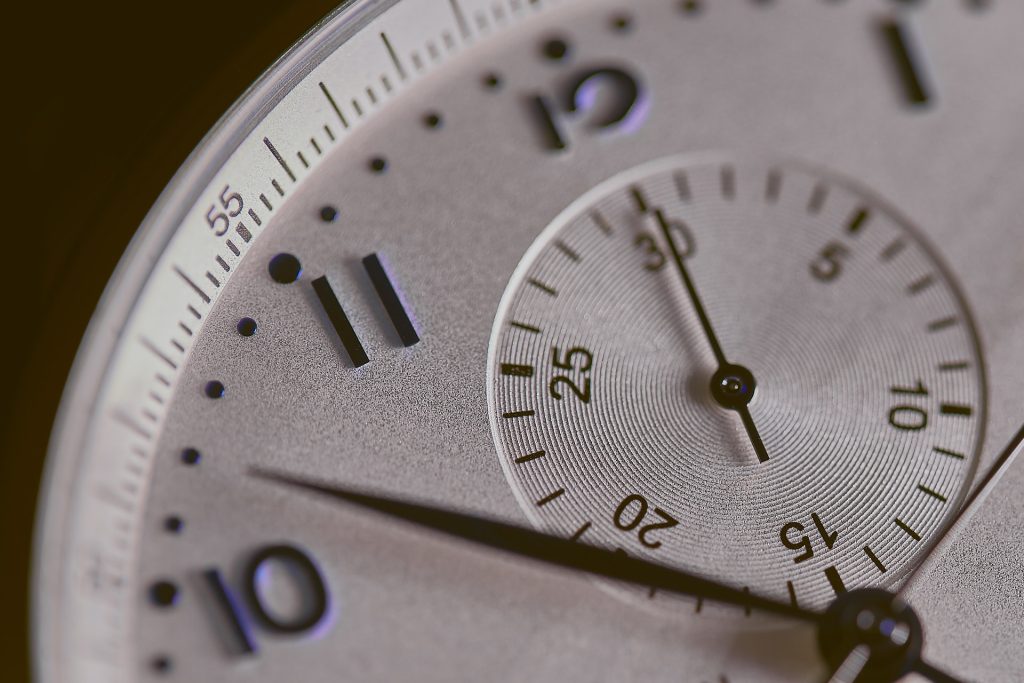
I don’t use 🍪 cookies anymore, I think they are too… rigid. I use localStorage
items. By default, they don’t expire and they are saved as a key/value pair. Here’s how to add an expiration date/time.
We will learn how to implement expiry times for items stored in the browser’s localStorage
.
This is how we set an item. We store the expected time of expiry along with the original information to be stored.
function setItemWithExpiry(key, value, ttl = 2592000000) {
const now = new Date();
// By default ttl (Time To Live) is 2592000000 (30 days)
// `item` is an object which contains the original value
// as well as the time when it's supposed to expire
const item = {
value: value,
expiry: now.getTime() + ttl
};
localStorage.setItem(key, JSON.stringify(item));
}
And this is how we get an item. When getting the item, we compare the current time with the stored expiry time. If the current time is greater than to stored expiry time, return null
and remove the item from storage.
function getItemWithExpiry(key) {
const itemStr = localStorage.getItem(key);
// If the item doesn't exist, return null
if (!itemStr) {
return null;
}
const item = JSON.parse(itemStr);
const now = new Date();
// Compare the expiry time of the item with the current time
if (now.getTime() > item.expiry) {
// If the item is expired, delete the item from storage
// and return null
localStorage.removeItem(key);
return null;
}
return item.value;
}
Enjoy!
Photo by Agê Barros on Unsplash.