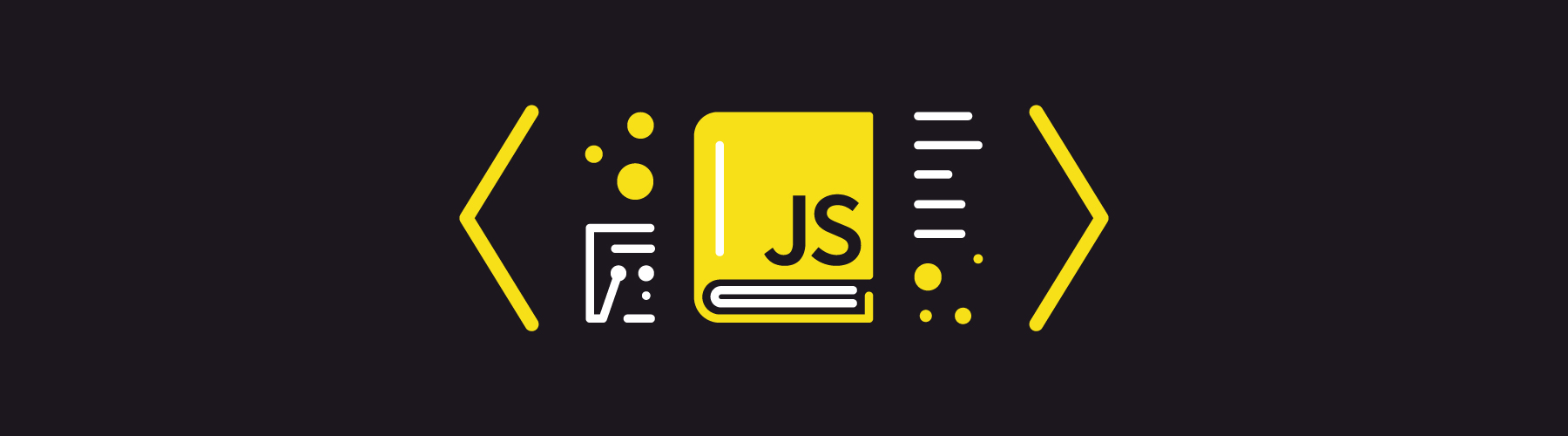
If you’ve been getting lots of email notices when using GitHub API calls related to:
Deprecation notice for authentication via URL query parameters
Please use the Authorization HTTP header instead, as using the access_token
query parameter is deprecated. If this token is being used by an app you don’t have control over, be aware that it may stop working as a result of this deprecation. Depending on your API usage, we’ll be sending you this email reminder on a monthly basis for each token and User-Agent used in API calls made on your behalf. Just one URL that was accessed with a token and User-Agent combination will be listed in the email reminder, not all.
Here’s what you need to know, when using access_token
as a query parameter, if you’re currently making an API call similar to:
curl "https://api.github.com/user/repos?access_token=my_access_token"
Instead, you should send the token in the header:
curl -H 'Authorization: token my_access_token' https://api.github.com/user/repos
When using PHP, here’s the correct approach:
<?php
// Switch to HTTP Basic Authentication for GitHub API v3
$curl = curl_init();
curl_setopt_array($curl, [
CURLOPT_URL => $requestUri,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => [
"Authorization: token " . $authorizeToken,
"User-Agent: YourAppName"
]
]);
$response = curl_exec($curl);
curl_close($curl);
Using JavaScript:
var data = null;
const xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.addEventListener("readystatechange", function () {
if (this.readyState === 4) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://api.github.com/repos/user/repo/releases");
xhr.setRequestHeader("Authorization", "token 1234567890");
xhr.setRequestHeader("User-Agent", "YourAppName");
xhr.setRequestHeader("Accept", "*/*");
xhr.setRequestHeader("Cache-Control", "no-cache");
xhr.setRequestHeader("Host", "api.github.com");
xhr.setRequestHeader("Accept-Encoding", "gzip, deflate");
xhr.setRequestHeader("Connection", "keep-alive");
xhr.setRequestHeader("cache-control", "no-cache");
xhr.send(data);
Or using the Fetch API:
var myHeaders = new Headers();
myHeaders.append("Authorization", "token 1234567890");
const requestOptions = {
method: 'GET',
headers: myHeaders,
redirect: 'follow'
};
fetch("https://api.github.com/repos/user/repo/releases", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
GitHub Authentication for WordPress Updater Solutions
If you are using GitHub to update your WordPress plugins (both public and private), switch to Andy Fragen’s solution. I have removed the GitHub updater from 10 plugins and 2 themes I am maintaining and switched to his GitHub Updater. I never looked back.
I wrote about this before, but maintaining a custom GitHub API solution is not worth it anymore.
Enjoy!