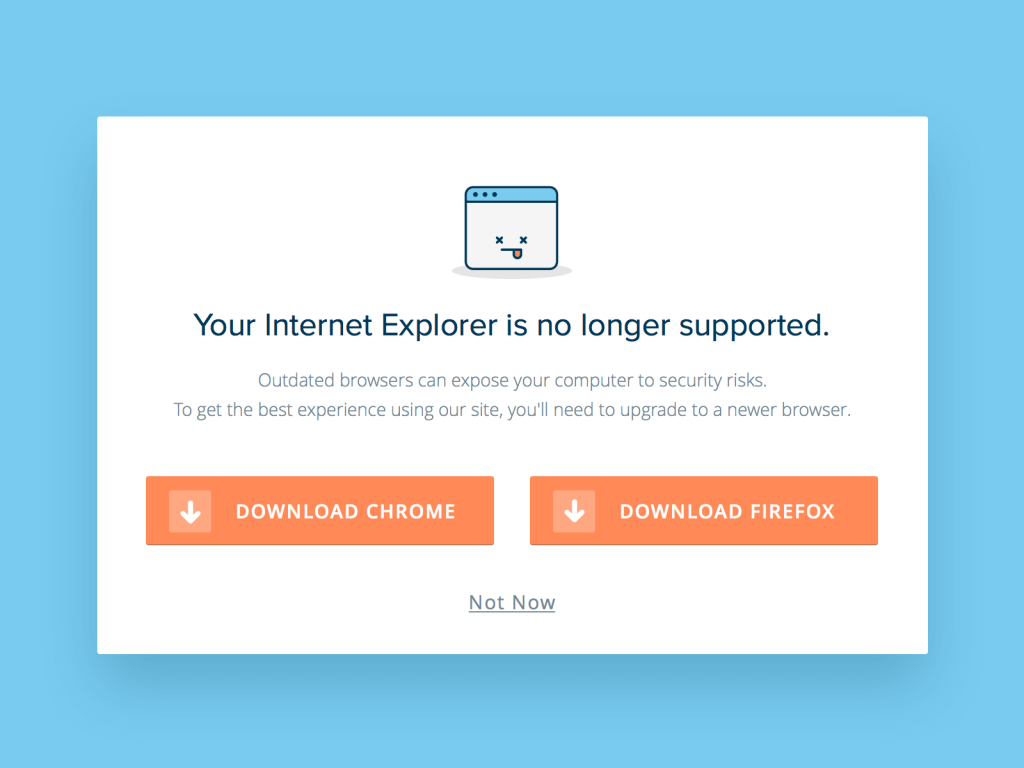
For years, I’ve relied on JavaScript and user agent detection to detect IE11 and below in order to show browser warnings. Here’s the code I’ve been using – an empty, hidden <div>
trigerrable via JavaScript.
HTML
<div class="unsupported-browser"></div>
CSS
.unsupported-browser {
display: none;
position: fixed;
z-index: 99999;
top: 16px;
left: 16px;
right: 16px;
background: #cf6a87;
color: #ffffff;
padding: 24px 16px;
text-align: center;
border-radius: 3px;
box-shadow: 0 0 48px rgba(0, 0, 0, 0.25);
}
JavaScript
var showBrowserAlert = (function () {
if (document.querySelector('.unsupported-browser')) {
var d = document.getElementsByClassName('unsupported-browser');
is_IE11 = isIE11();
if (is_IE11) {
d[0].innerHTML = '<b>Unsupported Browser!</b> This website will offer limited functionality in this browser. We only support the recent versions of major browsers like Chrome, Firefox, Safari, and Edge.';
d[0].style.display = 'block';
}
}
});
var isIE11 = (function() {
var isIE11 = !!window.MSInputMethodContext && !!document.documentMode;
// true on IE11, false on Edge and other IEs/browsers.
var ua = window.navigator.userAgent;
if (ua.indexOf("AppleWebKit") > 0) {
return false;
} else if (ua.indexOf("Lunascape") > 0) {
return false;
} else if (ua.indexOf("Sleipnir") > 0) {
return false;
}
array = /(msie|rv:?)\s?([\d\.]+)/.exec(ua);
version = (array) ? array[2] : '';
return (version == 11) ? true : false;
});
document.addEventListener('DOMContentLoaded', showBrowserAlert);
Note that the code is intentionally not ES6 to cater for legacy browsers.
Introducing Core Web Vitals
In the light of the recently introduced Core Web Vitals, I decided to save some script start-up speed and switch to PHP detection.
The actual detection code is way smaller and measurably faster, depending on the size of your JavaScript.
This script works with all IE versions, 8, 9, 10 and 11, and also with touchscreens.

PHP
if (preg_match('~MSIE|Internet Explorer~i', $_SERVER['HTTP_USER_AGENT']) || preg_match('~Trident/7.0(; Touch)?; rv:11.0~',$_SERVER['HTTP_USER_AGENT'])) {
echo '<div class="unsupported-browser"><b>Unsupported Browser!</b> This website will offer limited functionality in this browser. We only support the recent versions of major browsers like Chrome, Firefox, Safari, and Edge.</div>';
}
CSS
.unsupported-browser {
display: display;
position: fixed;
z-index: 99999;
top: 16px;
left: 16px;
right: 16px;
background: #cf6a87;
color: #ffffff;
padding: 24px 16px;
text-align: center;
border-radius: 3px;
box-shadow: 0 0 48px rgba(0, 0, 0, 0.25);
}
Using WordPress?
If you are using WordPress, use the wp_body_open
filter:
function custom_body_open() {
// Detect IE (IE11 or below)
if (preg_match('~MSIE|Internet Explorer~i', $_SERVER['HTTP_USER_AGENT']) || preg_match('~Trident/7.0(; Touch)?; rv:11.0~',$_SERVER['HTTP_USER_AGENT'])) {
echo '<div class="unsupported-browser"><b>Unsupported Browser!</b> This website will offer limited functionality in this browser. We only support the recent versions of major browsers like Chrome, Firefox, Safari, and Edge.</div>';
}
}
add_action('wp_body_open', 'custom_body_open');
Don’t forget to add the actual function right after the opening <body>
tag:
<body <?php body_class(); ?>>
<?php wp_body_open(); ?>
For more reading and advanced use of browser detection, you can learn more about an inbuilt PHP function – get_browser()
. Note that the get_browser()
function is slow and may affect web server performance if running on PHP5. It’s a bit better on PHP7, but still, I wouldn’t recommend it.