If you embed a Google Map iframe on your WordPress website, you may notice an increase in the total webpage size and a slowdown in your website’s loading speed. This can be addressed by implementing lazy loading for Google Maps.
In this article, I will show you to apply lazy loading to Google Map iframe code to improve the loading speed of your page.
Let’s say you have an embedded map:
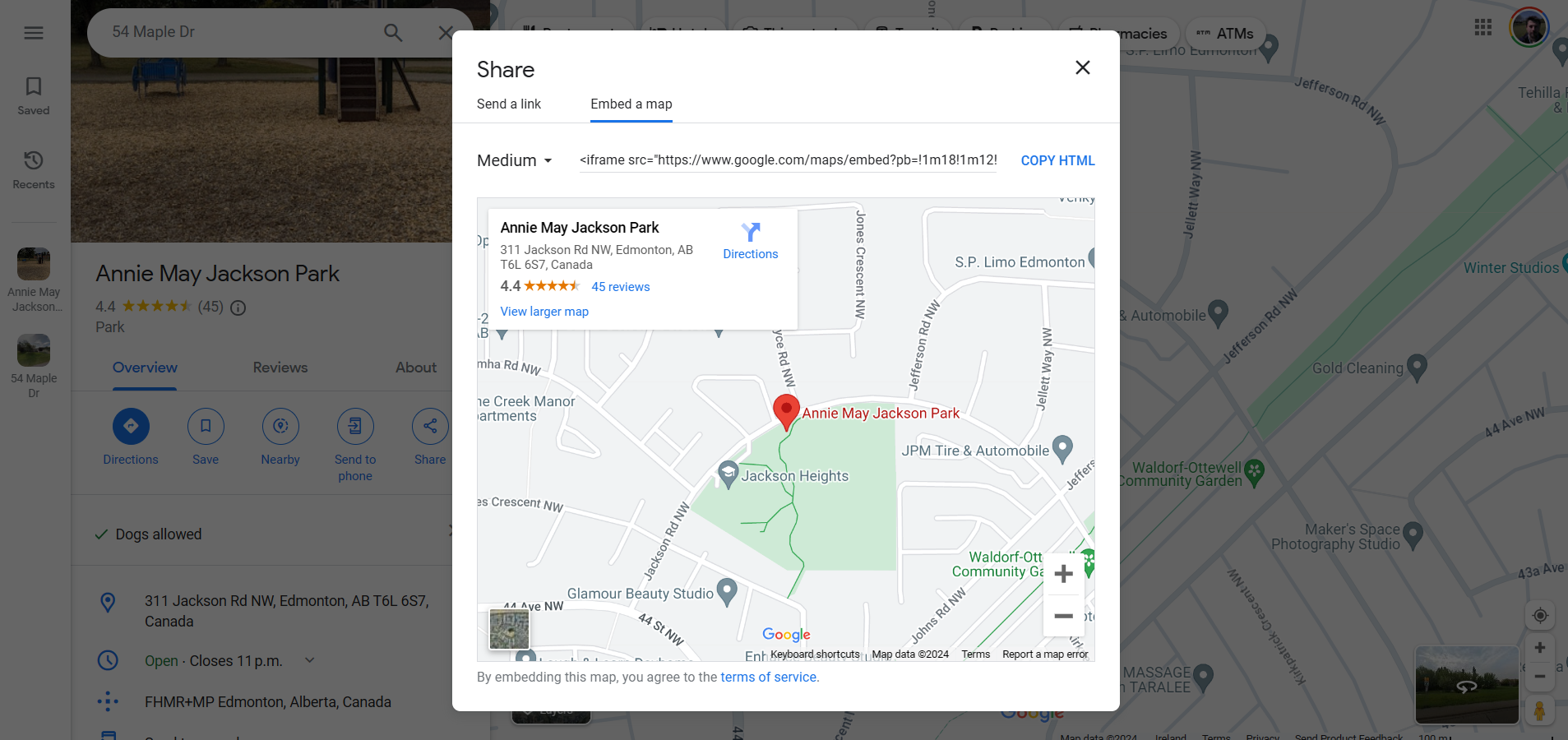
And you have the code below:
<iframe src="https://www.google.com/maps/embed?pb=!1m18!1m12!1m3!1d3373.978550513172!2d-113.40879688366614!3d53.48337304218447!2m3!1f0!2f0!3f0!3m2!1i1024!2i768!4f13.1!3m3!1m2!1s0x53a01855064a43c7%3A0x912b306bfe897bb!2sAnnie%20May%20Jackson%20Park!5e0!3m2!1sen!2sie!4v1714660542494!5m2!1sen!2sie" width="600" height="450" style="border:0;" allowfullscreen="" loading="lazy" referrerpolicy="no-referrer-when-downgrade"></iframe>
One thing you can try is to make sure you have the loading="lazy"
and defer
parameters in the iframe tag. However, this will not help a lot. So let’s make the iframe static.
We will change the tag from <iframe>
to <div>
, give it a class of lazy
, and replace the src
parameter with data-src
, like below:
<div class="lazy" data-src="https://www.google.com/maps/embed?pb=!1m18!1m12!1m3!1d3373.978550513172!2d-113.40879688366614!3d53.48337304218447!2m3!1f0!2f0!3f0!3m2!1i1024!2i768!4f13.1!3m3!1m2!1s0x53a01855064a43c7%3A0x912b306bfe897bb!2sAnnie%20May%20Jackson%20Park!5e0!3m2!1sen!2sie!4v1714660542494!5m2!1sen!2sie" width="600" height="450" style="border:0;" allowfullscreen="" loading="lazy" referrerpolicy="no-referrer-when-downgrade"></div>
Next, we need to style the <div>
element and only display it when it becomes visible (scrolled to) on screen:
<style>
.lazy {
position: relative;
width: 100%;
height: 450px;
overflow: hidden;
}
.lazy iframe {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
border: 0;
}
</style>
<script>
document.addEventListener("DOMContentLoaded", (event) => {
const observer = new IntersectionObserver((entries, observer) => {
entries.forEach((entry) => {
if (entry.isIntersecting) {
const iframe = document.createElement("iframe");
iframe.src = entry.target.dataset.src;
entry.target.appendChild(iframe);
observer.unobserve(entry.target);
}
});
});
const lazyLoadMapElements = document.querySelectorAll(".lazy");
lazyLoadMapElements.forEach((element) => {
observer.observe(element);
});
});
</script>
That’s it!
Now, if you have the map below the fold, it won’t load until you scroll and reach the <div>
element. This will give your pagespeed a boost, as it does not need to load the Google Maps assets and resources.
In my case, having a map in the footer saved me a lot of bandwidth, as most users would never reach the footer.
This is also useful if you are using a Google Maps API key, and you want to reduce your quota usage.