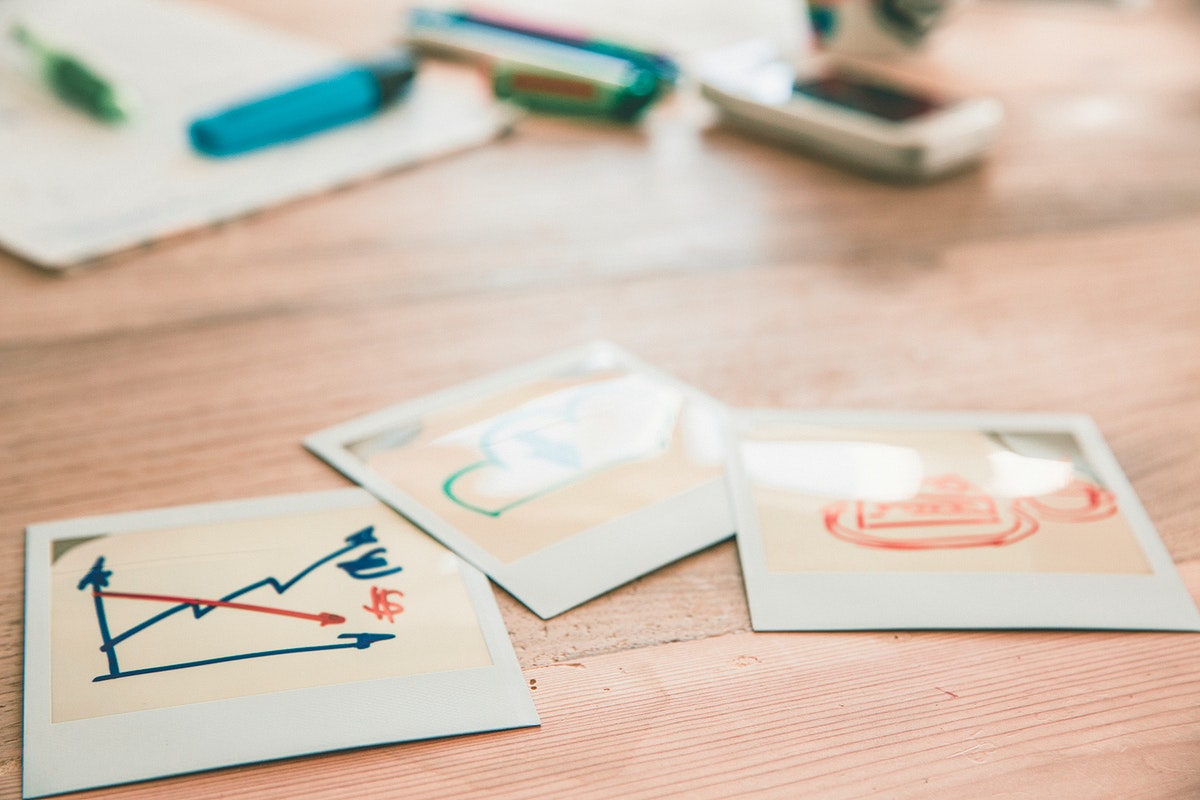
This small JavaScript snippet creates percentage graphs out of raw (array) data. Just input the name/value pairs of the involved graph elements, and leave the rest to the snippet. The values can be either absolute or percentage.
You can render more than one graph on the same page by invoking the graph function multiple times, with different array parameters.
Since the first example is created using absolute values, the script also displays the total value at the end.
What we will build
Here is a demo of both versions:
How to use
See below two versions of the JavaScript snippet, the first requiring that the graph values be absolute (e.g., 35
, 123
), while the second, in percentage (e.g., 25%
, 75%
).
Let’s add the required HTML elements:
<h3>This is an absolute values graph</h3>
<div id="graph-1"></div>
<h3>This is a percentage values graph</h3>
<div id="graph-2"></div>
Absolute Values Version
This version will automatically calculate percentages for all your values, based on the total number.
let graphx = [];
graphx[0] = ['Apples', 60];
graphx[1] = ['Pears', 75];
graphx[2] = ['Strawberries', 24];
graphx[3] = ['Bananas', 52];
function graphit(g, g_width, g_element) {
let total = 0,
calpercentage = 0,
calwidth = 0;
for (i = 0; i < g.length; i++) {
total += parseInt(g[i][1]);
}
let output = '<table border="0" cellspacing="0" cellpadding="8">';
for (i = 0; i < g.length; i++) {
calpercentage = Math.round((g[i][1] * 100) / total);
calwidth = Math.round(g_width * (calpercentage / 100));
output += `<tr><td>${g[i][0]}</td><td><svg xmlns="http://www.w3.org/2000/svg" width="${calwidth}" height="10"><g class="bar"><rect width="${calwidth}" height="10"></rect></g></svg> ${calpercentage}%</td></tr>`;
}
output += '</table>';
g_element.innerHTML = `${output}<br>Total fruits: <b>${total}</b>`;
}
graphit(graphx, 200, document.getElementById('graph-1')); // 200 is the maximum width of the graph, in pixels
Percentage Values Version
let graphv = [];
graphv[0] = ['Cranberries', 28];
graphv[1] = ['Redberries', 36];
graphv[2] = ['Blueberries', 30];
graphv[3] = ['Raspberries', 6];
graphv[4] = ['Strawberries', 80];
graphv[5] = ['Blackberries', 11];
graphv[6] = ['Lingonberries', 24];
graphv[7] = ['Cloudberries', 98];
function graph_it_percentage(g, g_width, g_element) {
let output = '<table border="0" cellspacing="0" cellpadding="8">',
calwidth_percentage = 0;
for (i = 0; i < g.length; i++) {
calwidth_percentage = g_width * (parseInt(g[i][1]) / 100);
output += `<tr><td>${g[i][0]}</td><td><svg xmlns="http://www.w3.org/2000/svg" width="${calwidth_percentage}" height="10"><g class="bar"><rect width="${calwidth_percentage}" height="10"></rect></g></svg> ${g[i][1]}%</td></tr>`;
}
output += '</table>';
g_element.innerHTML = output;
}
graph_it_percentage(graphv, 200, document.getElementById('graph-2')); // 200 is the maximum width of the graph, in pixels
Note that I have used an SVG for the bar, which can be easily coloured or styled as a gradient.